シンプルで効率的なコード短縮テクニック
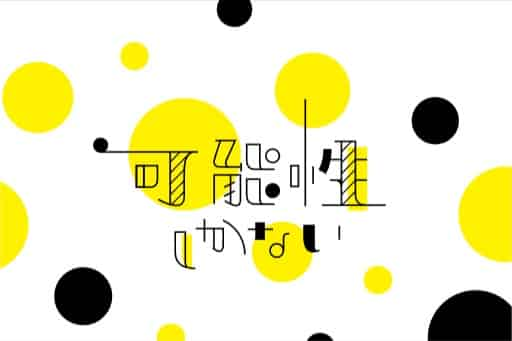
CSSは日々進化しています。
うまく使えば、便利にコードも少なく実装できますので、紹介していきます。
プロパティの省略
・margin
# Before
margin: 10px 0 10px 0;
# After
margin: 10px 0;
ージンのショートハンドでは、上下と左右が同じ値の場合に省略することができます。
・border
# Before
border-width: 1px;
border-style: solid;
border-color: #333;
# After
border: 1px solid #333;
borderプロパティで一行にまとめて指定可能です。
・transitionの利用
# Before
.element {
transition-property: all;
transition-duration: 0.3s;
transition-timing-function: ease-in-out;
}
# After
.element {
transition: all 0.3s ease-in-out;
}
ショートハンドで簡潔にアニメーションの指定ができます。
個別のトランスフォームプロパティ
# before
transform: translate(5rem);
transform: rotate(45deg);
transform: scale(2);
# after
translate: 5rem;
rotate: 45deg;
scale: 2;
display: noneの代替としてのvisibility: hidden
表示・非表示を切り替える場合、displayの代わりにvisibilityを使うことで、スペースを保持しつつ非表示にできます。
# Before
.hidden {
display: none;
}
# After
.hidden {
visibility: hidden;
}
要素を一時的に非表示にしつつ、レイアウトに影響を与えない場合は、visibility: hiddenが便利です。
CSS変数(Custom Properties)
:root {
--main-color: #3498db;
}
h1, p {
color: var(--main-color);
}
CSS変数は一度定義すればどこでも再利用でき、変更が容易になります。複数の箇所に同じスタイルを適用する際に非常に有効です。
calc() 関数
CSSでは、動的な計算をcalc()関数で簡単に行うことができます。
# Before
.width-half {
width: 50%;
padding: 10px;
}
# After
.width-half {
width: calc(50% - 20px);
}
calc()を使うことで、パーセンテージとピクセル値を組み合わせた動的な計算が可能です。これにより、より精密なレイアウト調整ができます。
clamp()関数でレスポンシブなフォントサイズ
# Before
h1 {
font-size: 2vw;
}
@media (min-width: 768px) {
h1 {
font-size: 24px;
}
}
@media (min-width: 1200px) {
h1 {
font-size: 32px;
}
}
# After
h1 {
font-size: clamp(16px, 4vw, 32px);
}
clamp()を使うと、フォントサイズが最小値・最大値の範囲内で可変となり、デバイスに応じたレスポンシブなスタイルが簡単に実現できます。
min()・max() 関数
# Before
max-width: 400px;
width: 100%;
# After
width: min(400px, 100%);
aspect-ratio プロパティ
aspect-ratioプロパティを使用することで、画像やビデオの縦横比を簡単に指定でき、従来のように余計なpaddingやpositionプロパティを使わずに済みます。
# Before
.image-container {
position: relative;
padding-bottom: 56.25%; /* 16:9 ratio */
height: 0;
}
.image-container img {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: 100%;
}
# After
.image-container {
aspect-ratio: 16 / 9;
}
inset
# Before
top: 0;
bottom: 0;
left: 0;
right: 0;
# After(要素は親要素の四辺にピタリと接するようになる)
inset: 0;
insetプロパティで上下左右の位置調整を一括で指定でき、コードが短くなります。
is()・where()関数でセレクタの簡略化
# Before
button:hover,
button:focus {
opacity: .5;
}
# After
button:is(:hover, :focus) {
opacity: .5;
}
:is() は、複数のセレクタをグループ化するための疑似クラスです。このグループに含まれるいずれかの条件が真であれば、そのスタイルが適用されます。以前は :matches() と呼ばれていましたが、現在は :is() が標準です。